Have you ever wanted to get custom notifications in Slack about new interactions in your GitHub repository? If so, then you're in luck. With the help of GitHub actions and Slack's Block Kit, it is super easy to set up automated workflows that will send custom messages to your Slack channel of choice. In this article, I will guide you on how to set up the Slack bot and send automatic messages using GH actions.
Create a Slack app
Firstly, we need to create a new Slack application. Go to Slack's app page. If you haven't created an app before you should see:
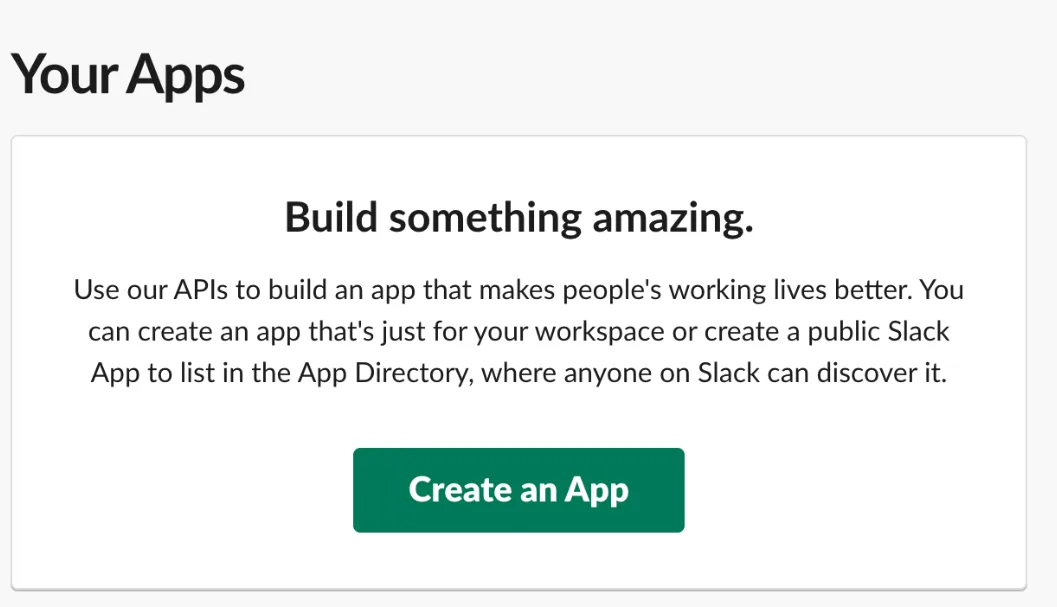
otherwise you might see a list of your existing apps:
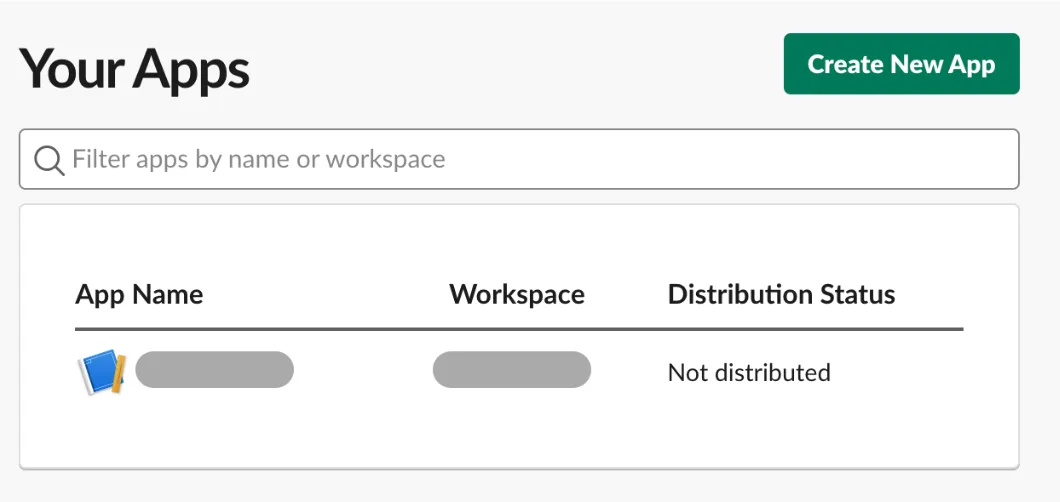
Let's click the Create an App
button. Frpm a modal that shows up, choose From scratch
option:
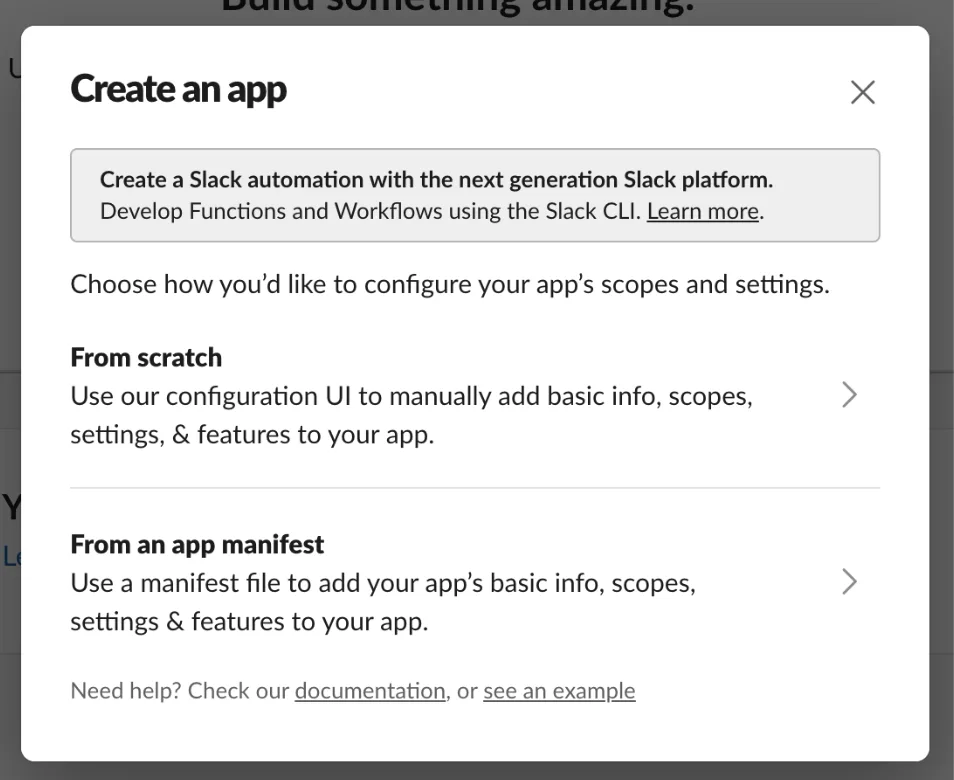
In the next step, we can choose the app's name (eg. My Awesome Slack App
) and pick a workspace that you want to use for testing the app.
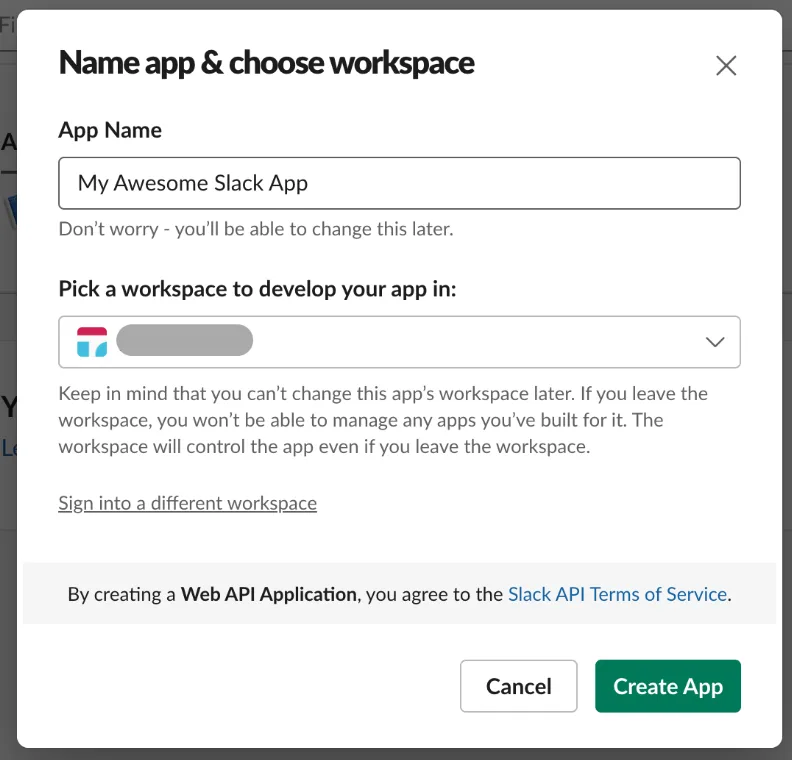
After the app is created successfully, we need to configure a couple of additional options. Firstly we need to configure the OAuth & Permissions
section:
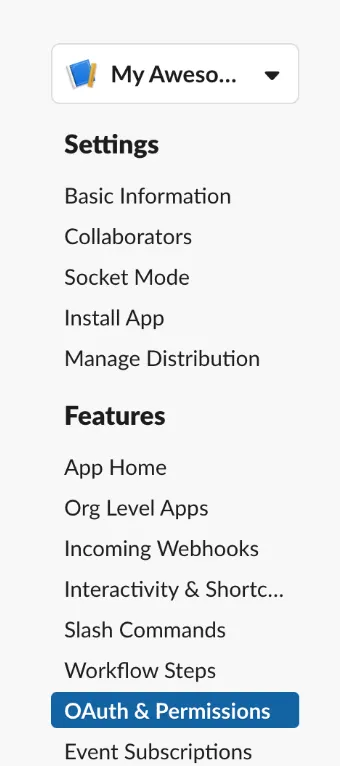
In the Scopes
section, we need to add a proper scope for our bot. Let's click Add an OAuth Scope
in the Bot Token Scopes
section, and select an incoming-webhook
scope:
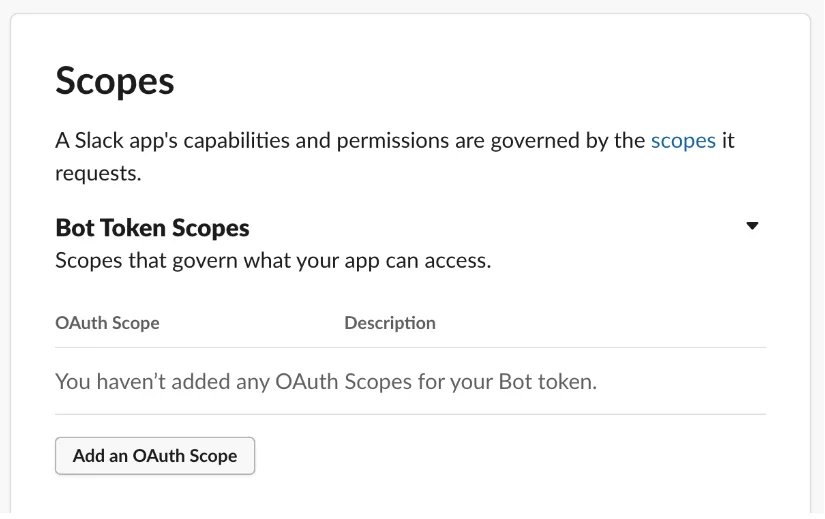
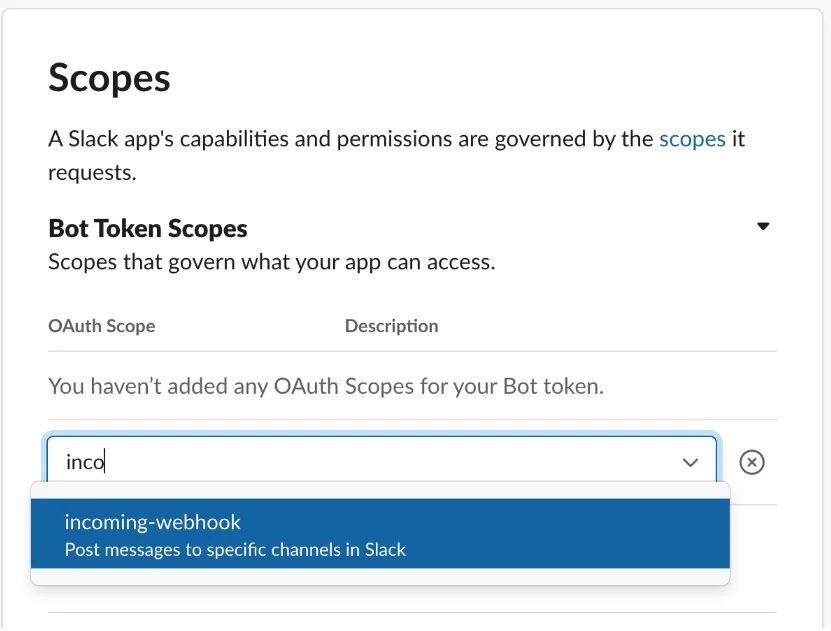
Next, in OAuth Tokens for Your Workspace
section, click Install to Workspace
and choose a channel that you want messages to be posted to.
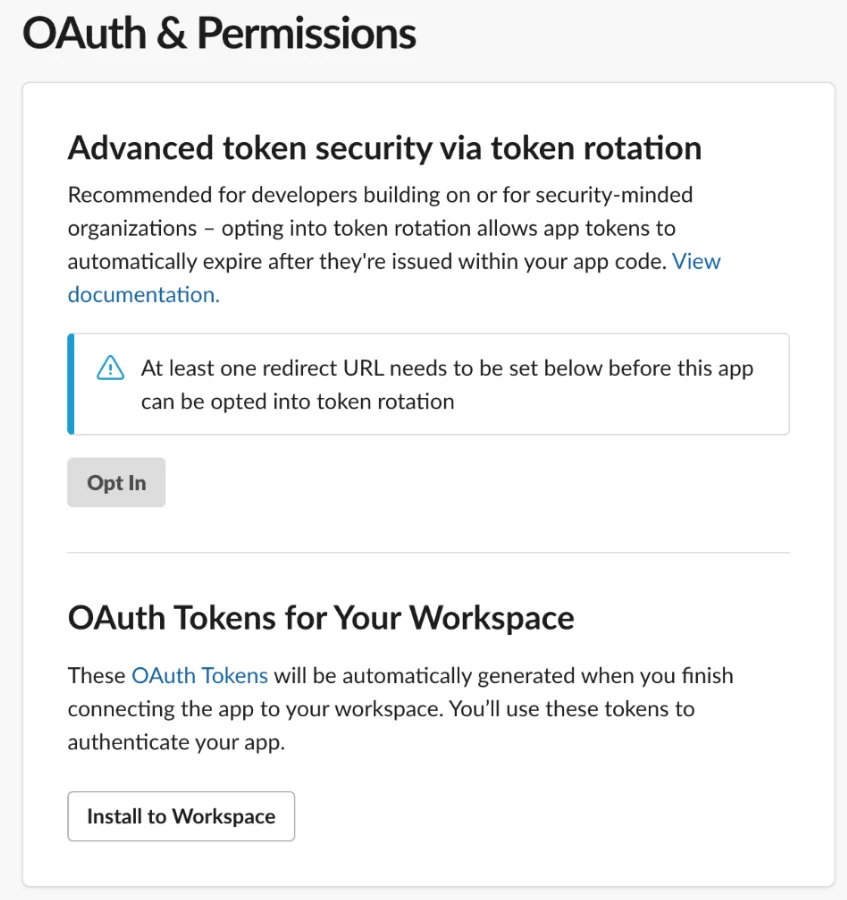
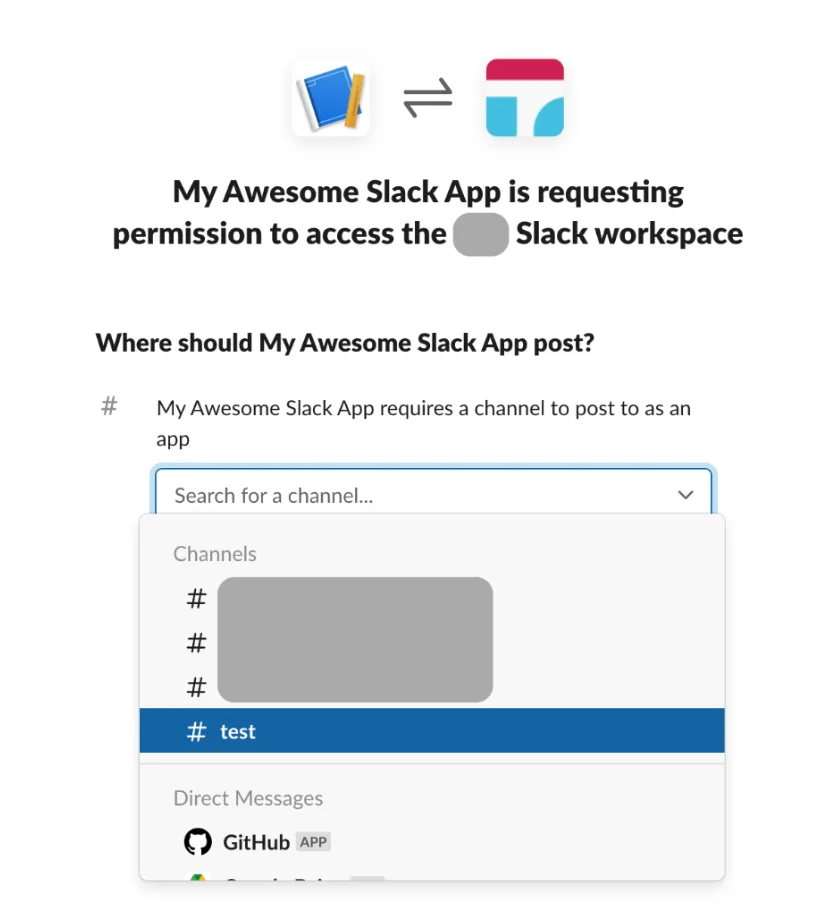
Finally, let's go to Incoming Webhooks page
, and activate the incoming hooks toggle (if it wasn't already activated).
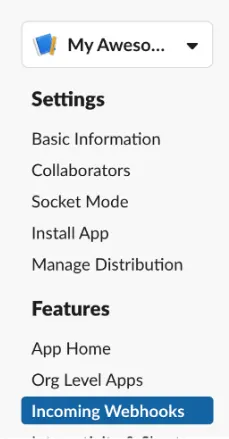
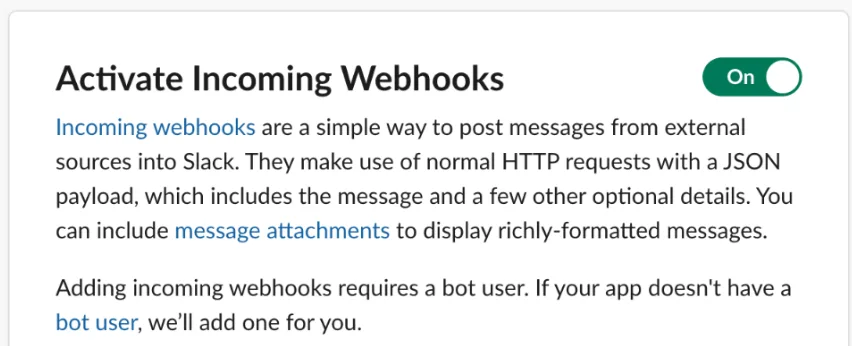
Copy the webhook URL (we will need it for our GitHub action).
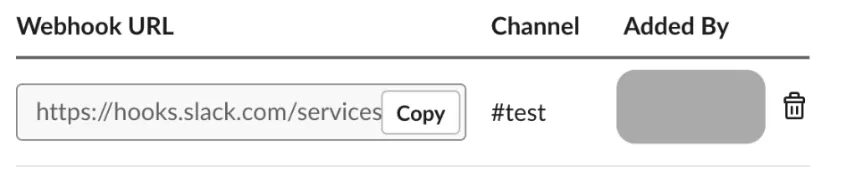
Create a Github Action Workflow
In this section, we will focus on setting up the GitHub action workflow that will post messages on behalf of the app we've just created. You can use any of your existing repositories, or create a new one.
Setting Up Secrets
In your repository, go to Settings
-> Secrets and variables
-> Actions
section and create a New Repository Secret
.
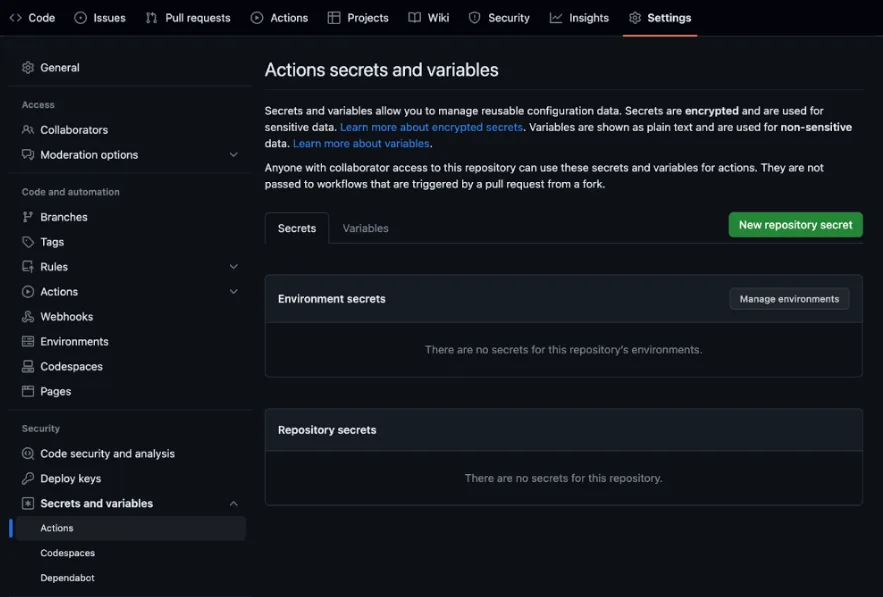
We will call the secret SLACK_WEBHOOK_URL
and paste the url we've previously copied as a value.
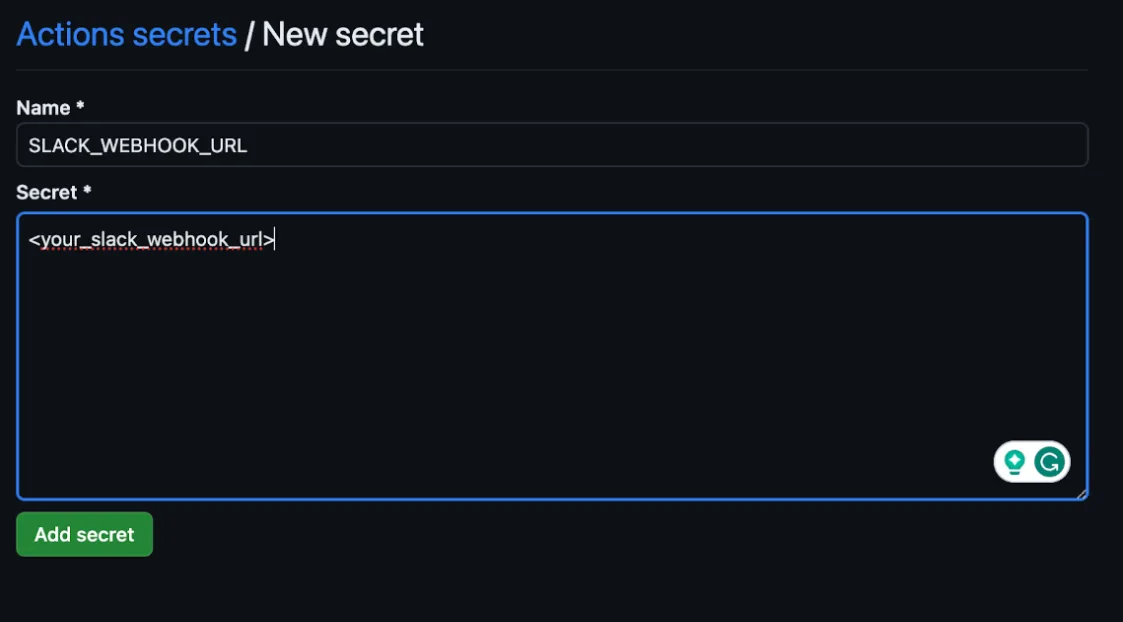
Create a workflow
To actually send a message we can use slackapi/slack-github-action GitHub action. To get started, we need to create a workflow file in .github/workflows
directory. Let's create .github/workflows/slack-message.yml
file to your repository with the following content and commit the changes to main
branch.
name: 'Send Slack notification'
on:
workflow_dispatch:
jobs:
send-slack-notification:
runs-on: ubuntu-latest
steps:
- name: Send message to Slack workflow
id: slack
uses: slackapi/slack-github-action@v1.24.0
with:
payload: |
{
"text": "Hello Slack",
"blocks": [
{
"type": "section",
"text": {
"type": "mrkdwn",
"text": "GitHub Action (${{ github.run_id }}) posted this message"
}
}
]
}
env:
SLACK_WEBHOOK_URL: ${{ secrets.SLACK_WEBHOOK_URL }}
SLACK_WEBHOOK_TYPE: INCOMING_WEBHOOK
In this workflow, we've created a job that uses slackapi/slack-github-action
action and sends a basic message with an action run id. The important thing is that we need to set our webhook url as an env variable. This was the action can use it to send a message to the correct endpoint.
We've configured the action so that it can be triggered manually. Let's trigger it by going to Actions
-> Send Slack notification
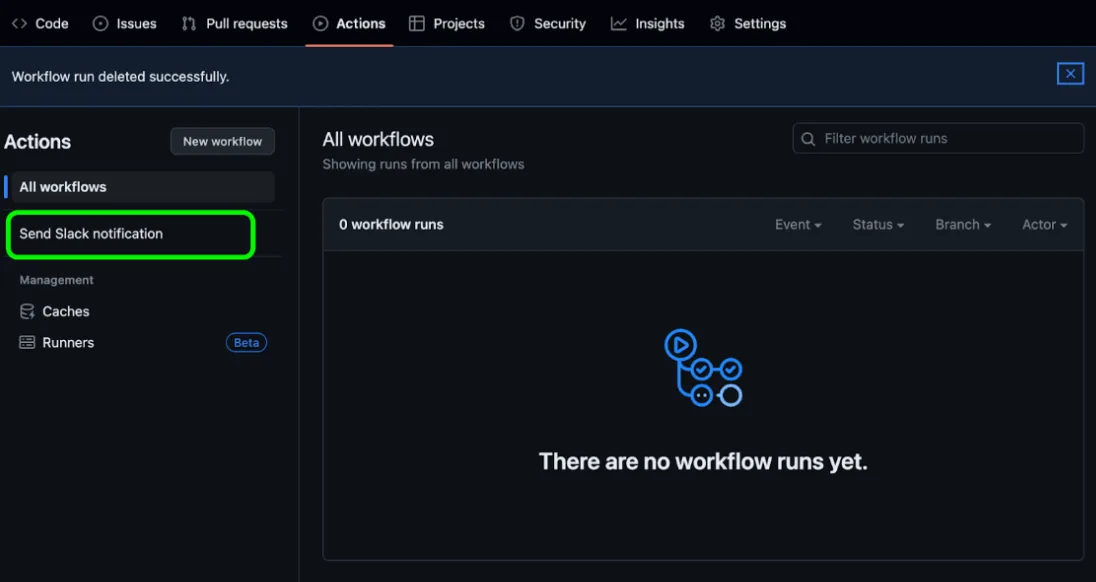
We can run the workflow manually in the top right corner.
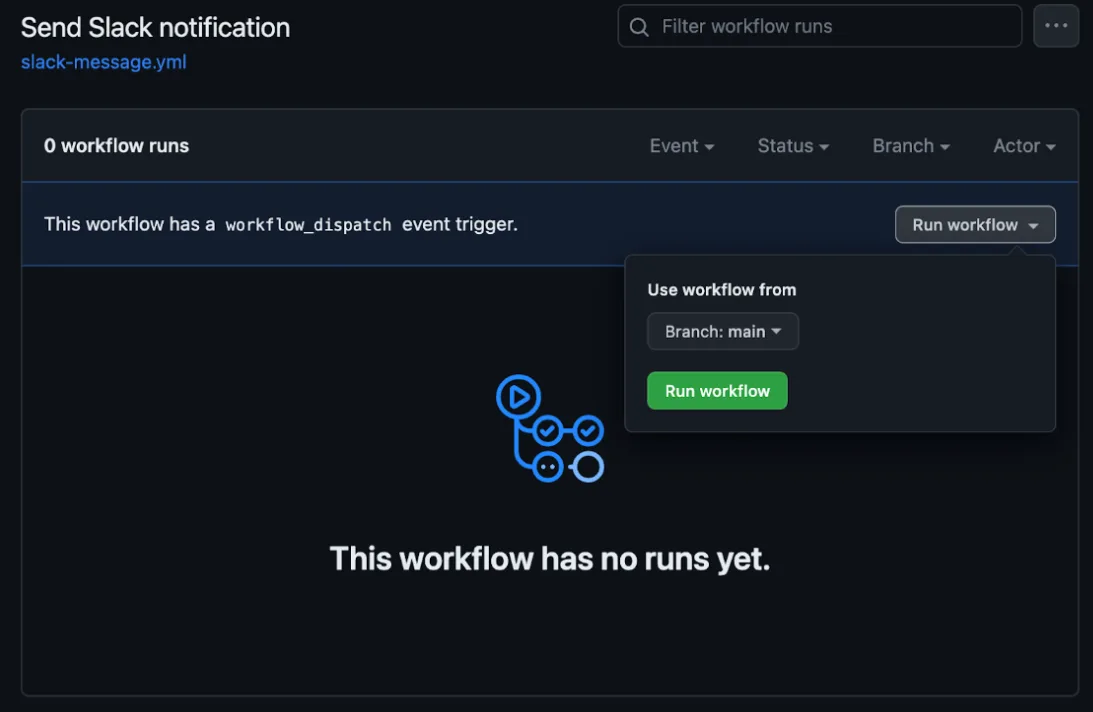
After running the workflow, we should see our first message in the Slack channel that we've configured earlier.

Manually triggering the workflow to send a message is not very useful. However, we now have the basics to create more useful actions.
Automatic message on pull request merge
Let's create an action that will send a notification to Slack about a new contribution to our repository. We will use Slack's Block Kit to construct our message.
Firstly, we need to modify our workflow so that instead of being manually triggered, it runs automatically when a pull requests to main
branch is merged. This can be configured in the on
section of the workflow file:
on:
pull_request:
branches:
- main
types:
- closed
Secondly, let's make sure that we only run the workflow when a pull request is merged and not eg. closed without merging. We can configure that by using if
condition on the job
:
- name: Send message to Slack workflow
id: slack
uses: slackapi/slack-github-action@v1.24.0
with:
payload: |
{
"blocks": [
{
"type": "header",
"text": {
"type": "plain_text",
"text": "A PR was just merged into ${{ github.repo }} :tada:",
"emoji": true
}
},
{
"type": "divider"
},
{
"type": "section",
"text": {
"type": "mrkdwn",
"text": "*${{ github.event.pull_request.user.login }}* has just contributed to ${{ github.repository }} :partying_face:"
}
}
]
}
We've used a repository name (github.repository
) as well as the user login that created a pull request (github.event.pull_request.user.login
), but we could customize the message with as many information as we can find in the pull_request
event. If you want to quickly edit and preview the message template, you can use the Slack's Block Kit Builder.
Now we can create any PR, eg. add some changes to README.md, and after the PR is merged, we will get a Slack message like this.

Summary
As I have shown in this article, sending Slack messages automatically using GitHub actions is quite easy. If you want to check the real life example, visit the starter.dev project where we are using the slackapi/slack-github-action
to get notifications about new contributions (send-slack-notification.yml)
If you have any questions, you can always Tweet or DM me at @ktrz. I'm always happy to help!